Appearance
合并两个有序链表
简单
将两个升序链表合并为一个新的 升序 链表并返回。新链表是通过拼接给定的两个链表的所有节点组成的。
示例 1:
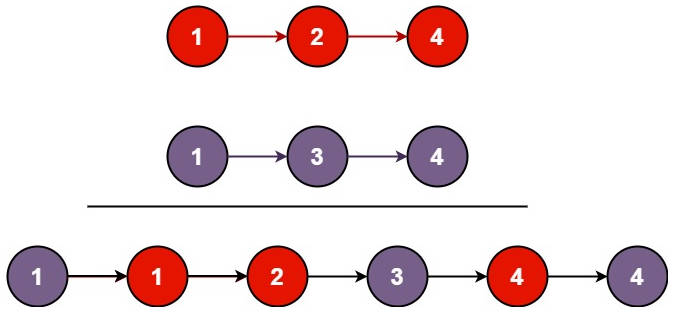
输入:l1 = [1,2,4], l2 = [1,3,4]
输出:[1,1,2,3,4,4]
示例 2:
输入:l1 = [], l2 = []
输出:[]
示例 3:
输入:l1 = [], l2 = [0]
输出:[0]
提示:
- 两个链表的节点数目范围是
[0, 50]
-100 <= Node.val <= 100
l1
和l2
均按 非递减顺序 排列
题解
1.迭代+哨兵节点
解题思路
- 定义一个哨兵节点 dummy,使用 dummy.next 来保存需要返回的头节点
- 判断 l1 和 l2 哪个更小,就把这个节点接到 dummy 下一个
- 直到有一边为 null,即可将另一边剩余的都接到 dummy 上
javascript
var mergeTwoLists = function (list1, list2) {
let dmy = new ListNode(0, null);
let curr = dmy;
while (list1 && list2) {
if (list1.val < list2.val) {
curr.next = list1;
list1 = list1.next;
} else {
curr.next = list2;
list2 = list2.next;
}
curr = curr.next;
}
if (list1 === null) curr.next = list2;
if (list2 === null) curr.next = list1;
return dmy.next;
};
2.递归
解题思路
- 递归边界:如果其中一个链表为空,直接返回另一个链表作为合并后的结果;
- 如果两个链表都不为空,则比较两个链表当前节点的值,并选择较小的节点作为新链表的当前节点;例如 list1 的节点值更小,那么递归调用 mergeTwoLists(list1.next, list2),将递归返回的链表接在 list1 的末尾;
javascript
var mergeTwoLists = function (list1, list2) {
if (list1 === null) return list2;
if (list2 === null) return list1;
if (list1.val < list2.val) {
list1.next = mergeTwoLists(list1.next, list2);
return list1;
}
list2.next = mergeTwoLists(list1, list2.next);
return list2;
};