Appearance
索引访问类型
我们可以使用一个索引访问类型来查询另一个类型上的特定属性:
typeScript
type Person = { age: number; name: string; alive: boolean };
type Age = Person["age"];
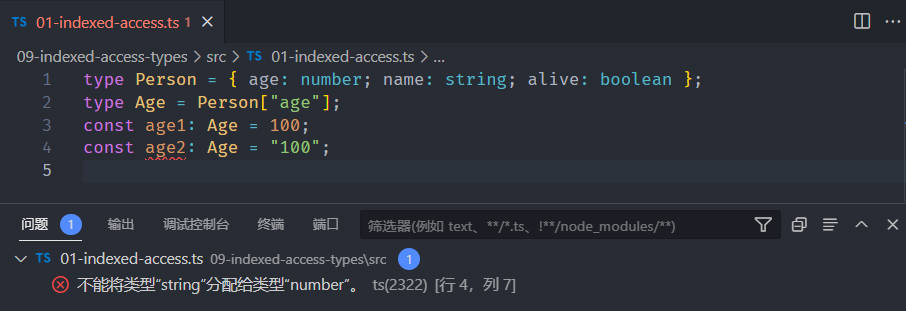
索引类型本身就是一个类型,所以我们可以完全使用 unions、 keyof 或者其他类型。
typeScript
interface Person {
name: string
age: number
alive: boolean
}
// type I1 = string | number
type I1 = Person["age" | "name"];
const i11:I1 = 100
const i12:I1 = ''
// type I2 = string | number | boolean
type I2 = Person[keyof Person];
const i21:I2 = ''
const i22:I2 = 100
const i23:I2 = false
// type I3 = Person[AliveOrName];
type AliveOrName = "alive" | "name";
const aon1:AliveOrName = 'alive'
const aon2:AliveOrName = 'name'
如果你试图索引一个不存在的属性,你甚至会看到一个错误:
typescript
type I1 = Person["alve"];
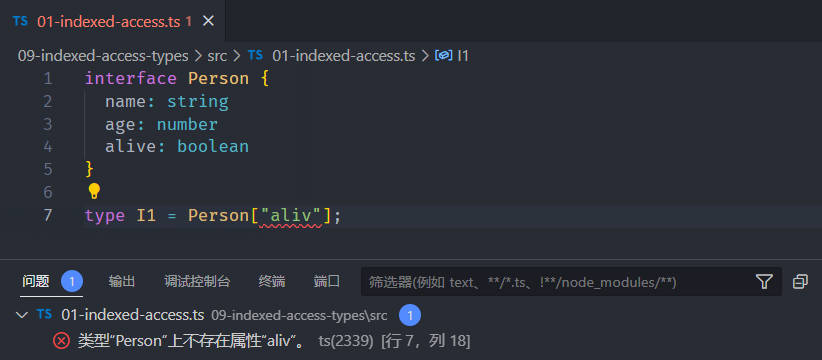
另一个使用任意类型进行索引的例子是使用 number 来获取一个数组元素的类型。我们可以把它和 typeof 结合起来,方便地获取一个数组字面的元素类型。
typescript
const MyArray = [
{ name: "Alice", age: 15 },
{ name: "Bob", age: 23 },
{ name: "Eve", age: 38 },
];
/* type Person = {
name: string;
age: number;
} */
type Person = (typeof MyArray)[number];
const p: Person = {
name: "xiaoqian",
age: 11,
};
// type Age = number
type Age = (typeof MyArray)[number]["age"];
const age: Age = 11;
// 或者
// type Age2 = number
type Age2 = Person["age"];
const age2: Age2 = 11;
你只能在索引时使用类型,这意味着你不能使用 const 来做一个变量引用:
typescript
const key = "age";
type Age = Person[key];
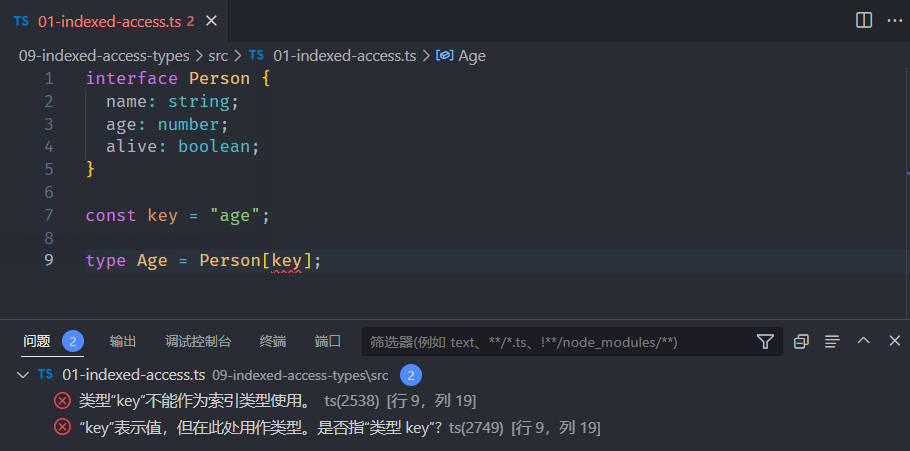
然而,你可以使用类型别名来实现类似的重构风格:
typescript
type key = "age";
type Age = Person[key];